Using the Wget Linux command, it is possible to download an entire website, including all assets and scripts. It is occasionally necessary to download and archive a large site for local viewing, and Wget makes this an easy process. more…
Exposing Configuration in Symfony 2 Bundles
In Symfony 2 there are two main ways to define configuration options for a bundle. The first way is to simply define parameters in the service container and the second is to expose semantic configuration for your bundle. more…
Unvalidated Redirects and Forwards Security Vulnerabilities in PHP
Unvalidated redirects and forwards are when an application uses untrusted data to redirect a user to a new webpage. This poses a security threat since an attacker can use the application to redirect unsuspecting users to a malicious site in a phishing scam. more…
Preventing SQL Injection in PHP
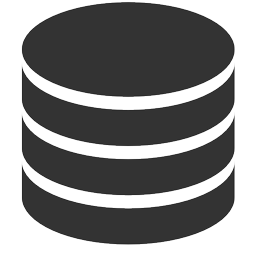
SQL Injection is a vulnerability that allows an attacker to insert or inject a SQL query into an application. Injection is number one vulnerability on the OWASP Top Ten list for 2013. Common ways to exploit this vulnerability is to add a SQL statement into a form element or by sending POST/GET requests with the query using known parameters. The risk of SQL injection is loss or compromise of critical or sensitive data. more…
Bash Script to Create Apache Virtual Hosts
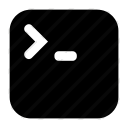
Creating a new Apache virtual host is something that’s done for every web project. It’s not a complicated process, but it can be simplified further by creating a bash script to automate the process. My script will update the Apache vhosts, add the new site to the hosts file, create the site directory if it doesn’t exist, and restart Apache. more…
Managing the iOS Network Activity Indicator

The network activity indicator in iOS is the spinning wheel in the upper left of the screen in the status bar next to the carrier name and connection strength meters. The indicator spins when the device is using the network offering the user great feedback when they are using data. more…
Get First n Items of NSArray in Objective C, or any range.
If you need to get the first n items in an NSArray in Objective C then you can use the subarrayWithRange: method. This method will work to get any range of items of an array and will return a new array containing those items. For example, to get the first 20 elements of the NSArray myArray, try this:
NSArray *firstTwentyItems = [myArray subArrayWithRange:NSMakeRange(0, MIN(20, [myArray count])];
To get the last 20 elements simply update the range like this:
NSArray *lastTwentyItems = [myArray subArrayWithRange:NSMakeRange(MAX(0, ([myArray count] - 20)), [myArray count])];
Using the MIN() and MAX() macros help ensure the range is within the bounds of the NSArray.
Include Javascript or CSS to a Drupal Form using #attached
If you’re creating a form using Drupal and find you want to add some javascript or CSS then you can use the “#attached” attribute to do so. Using “#attached” you can include local javascript/CSS files, external javascript/CSS files, or inline styles and scripts. more…
Order MySQL Query by User Defined Order Using the Field() Function
I was writing a MySQL database query and ran into an interesting challenge where my result set needed to be ordered in a predefined order to meet business logic requirements. Typically the ORDER BY clause will sort using numeric or alphabetical order. This is pretty limiting if you want to define an enumeration of values that should provide the sort order. more…
Update a Drupal Module’s Database Schema Using hook_update_N
Drupal allows a module developer to easily define a database schema using hook_schema but this won’t work if you need to update the database schema during an update to the module. Fortunately, there is a nice hook in Drupal called hook_update_N that you can place in your module’s .install file. more…